Mailgun
Send an email to a user.
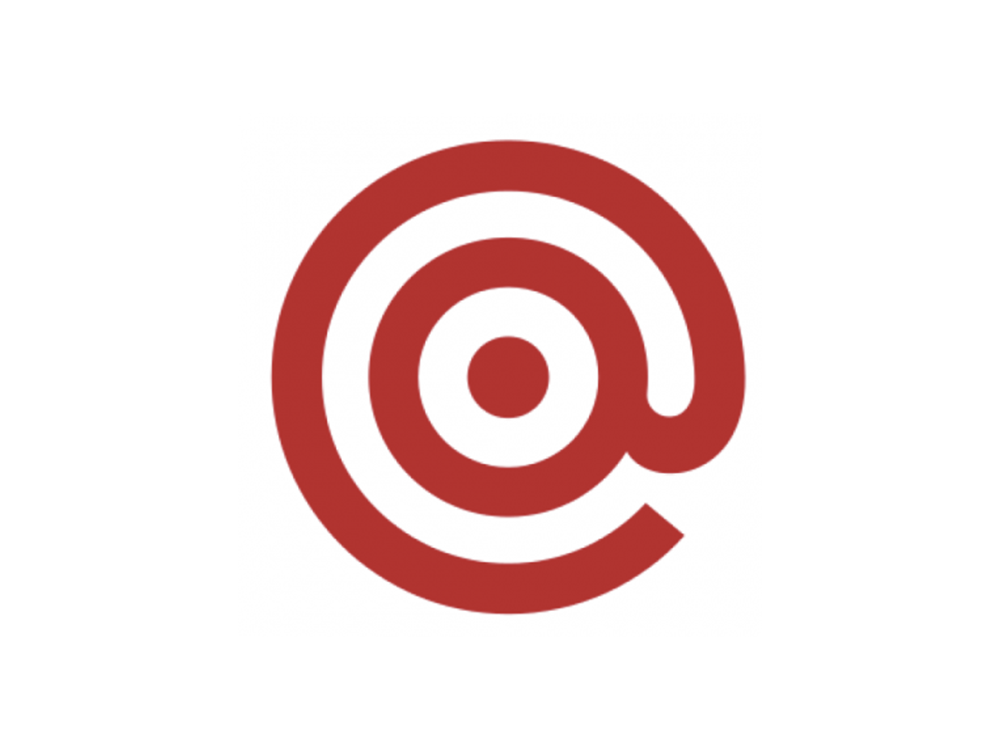
const mailgunSdk = require('mailgun-js');
const apiKey = process.env.MAILGUN_API_KEY;
const domain = `mail.${process.env.DOMAIN}`;
const mailgun = mailgunSdk({ apiKey, domain });
export default async (req, res) => {
let response;
try {
response = await mailgun.messages().send({
to: req.body.to,
from: req.body.from,
subject: req.body.subject,
text: req.body.text
});
} catch (error) {
return res.status(error.statusCode || 500).json({ error: error.message });
}
return res.status(200).json({ result: response.message });
};
Usage
1 Create Mailgun Account
First, create a Mailgun account. Then, retrieve your API key.
2 Add & Verify Domain
If you want emails to come from your email address, you'll need to add a domain. Don't want this right now? Use the sandbox domain.
3 Add Environment Variables
To securely access the API, we need to include the secret with each request. We also do not want to commit secrets to git. Thus, we should use an environment variable.